We all use Lerp
, but there are very few things we actually want to interpolate linearly in games. Of
course, we use easings curves.
Although Unity's Animation Curves could be used, sometimes you just want a fast implementation that would save you from creating another field.
This is where Easings
comes in. It's a library of curves that can be used to interpolate between two
values in a clean and fast way.
The library contains most of the popular easing curves in use, most being listed on Easings.net:
Linear
InQuad
OutQuad
InOutQuad
InCubic
OutCubic
InOutCubic
InQuart
OutQuart
InOutQuart
InQuint
OutQuint
InOutQuint
InSine
OutSine
InOutSine
InExpo
OutExpo
InOutExpo
InCirc
OutCirc
InOutCirc
InElastic
OutElastic
InOutElastic
InBack
OutBack
InOutBack
InBounce
OutBounce
InOutBounce
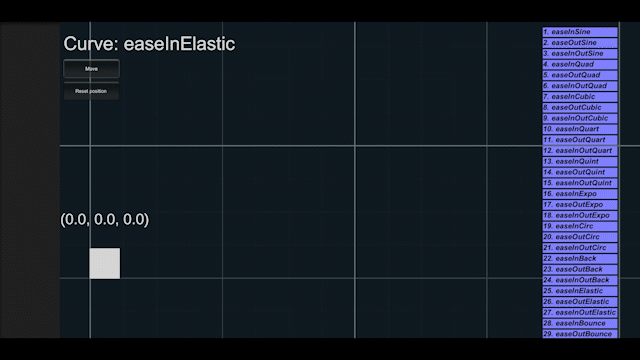
A very simple example of practical use:
using UnityEngine;
public class Example : MonoBehaviour
{
void Update()
{
var x = Mathf.Lerp(0, 10, Easings.InOutQuad(t));
}
}
All of the curves were thoroughly tested on Desmos Graph.
The entirety of this system is publicly available on Github.
Lastly, the system is bundled as a Unity custom package, making it easy to update.